Flutter をモーダルダイアログ表示させる方法についてまとめていきます。 現在の画面に小画面を表示します。モーダル表示は小画面を閉じるまで他の操作ができない画面です。
Flutter でのモーダル表示には、マテリアルの showDialog や showModalBottomSheet があります。
showDialog でモーダル表示
showDialog function - material library - Dart API
API docs for the showDialog function from the material library, for the Dart programming language.モーダルを表示する
ボタンを押すと、モーダルが表示されます。
例はオーバーレイとテキストが表示しています。
モーダルの中には自由にウィジェットを配置できます。
class ShowModalPage extends StatelessWidget {
const ShowModalPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: ElevatedButton(
child: const Text('モーダルを表示する'),
onPressed: () {
showDialog<void>(
context: context,
builder: (BuildContext context) {
return const Center(
child: Text(
'モーダルコンテンツ',
style: TextStyle( // デフォルトのテキストスタイルが派手すぎるので、スタイルを指定しています。
color: Colors.white,
decoration: TextDecoration.none,
fontSize: 40.0,
),
),
);
},
);
},
),
),
);
}
}
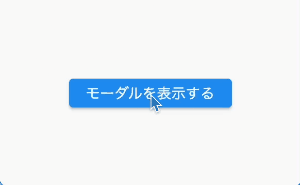
showModalBottomSheet でモーダル表示
showModalBottomSheet function - material library - Dart API
API docs for the showModalBottomSheet function from the material library, for the Dart programming language.モーダルを表示する
ボタンを押すと、モーダルが表示されます。
showModalBottomSheet は小画面を画面下部から表示します。
class ShowModalBottomSheetPage extends StatelessWidget {
const ShowModalBottomSheetPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: ElevatedButton(
child: const Text('モーダルを表示する'),
onPressed: () {
showModalBottomSheet<void>(
context: context,
builder: (BuildContext context) {
return const Center(
child: Text(
'モーダルコンテンツ',
),
);
},
);
},
),
),
);
}
}
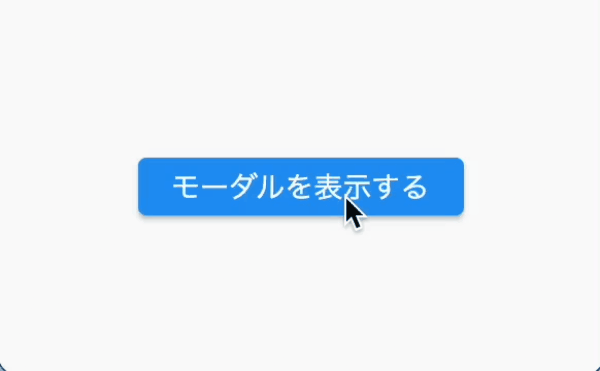
ModalBottomSheet を閉じる
showModalBottomSheet で表示したモーダルを閉じるには、Navigator.pop を使います。
TextButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('閉じる'),
),